Inheriting from another smart contract: Understanding storage in Ethereum
When writing smart contracts on the Ethereum blockchain, one of the fundamental concepts to understand is inheritance. Inheritance allows a new contract (the inheritor) to build upon or modify the functionality of an existing contract (the parent). However, there is one crucial aspect to keep in mind when working with storage: the this
keyword and its implications.
In your example, contract A has an “immutable” variable “x”, initialized with the value 42. In contract B, which inherits from contract A, it is not explicitly specified that “y” should be inherited or have a specific value. However, we will explore why y
might appear to be set to 0 in Contract B.
Why does y
not inherit the value of x
from Contract A?
In Ethereum contracts, when the “immutable” keyword is used, non-immutable variables (such as constants) are not affected by inheritance. This is because immutability is a contract-level property that prevents modification after the constructor call.
The reason you might see “y” set to 0 in Contract B is due to the way Ethereum storage works for inherited variables:
- When a new contract inherits from an existing one, it uses the storage location of its parent (Contract A) as its own.
- If a variable is not initialized with a specific value in the constructor call, it will be initialized with the default value of the parent contract’s storage location.
- Since
y
is not explicitly initialized with a value in the constructor call of Contract B, it defaults to 0.
Workarounds: Assigning a value to y
To work around this problem, you have a couple of options:
- Use an explicit variable declaration: In your code, you can declare
y
as an instance variable, like this:
“solidity
contract A {
immutable uint x;
constructor() {
x = 42;
y = 0; // Assign a value to y explicitly
}
…
}
Use the “memory” storage type
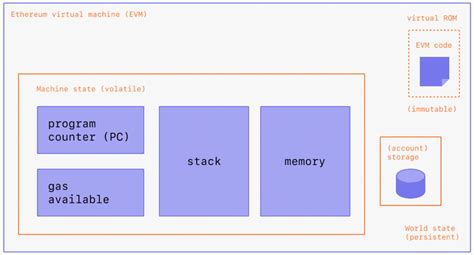
: When working with smart contracts, it is often more convenient to use the “memory” storage type instead of “storage”. The “memory” type allows you to dynamically allocate memory and deallocate it as needed.
"solidity
contract A {
immutable uint x;
public constructor() {
x = 42;
}
...
}
Contract B extends A {
public function setY(uint y) {
// y is now accessible in contract B
}
}
In this example, y
can be modified directly without affecting inheritance.